Hey Guys, Today we are going talk about using Sqlite with Godot! First why would I want to use SQLite and what is SQLite? SQLite is a small embeded database that allows the user to store data and query on it.
Ok that’s great why do I want to use it.
The nice thing about Sqlite is the ability to have all of your data in one area. The ability to query it is the most important part with that you can get your data in the simplest way.
Open up Godot and go ahead and create your project.
Setting Up Our Database
To set up our database lets go ahead and download DB Browser for SQLite. This tool will help us admin our database by giving us a gui in which we can create and edit our database!
Lets go ahead and create a database so lets click
New database
Lets choose where we want our database to go, so lets go out to our project create a folder and name it datastore. Let name our database data.
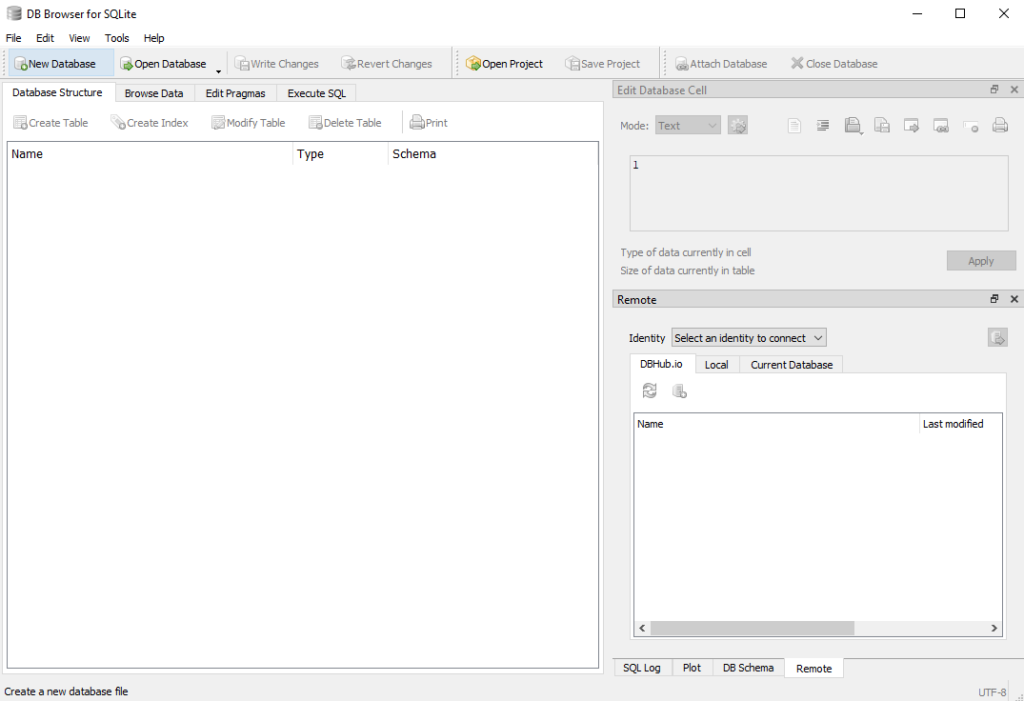
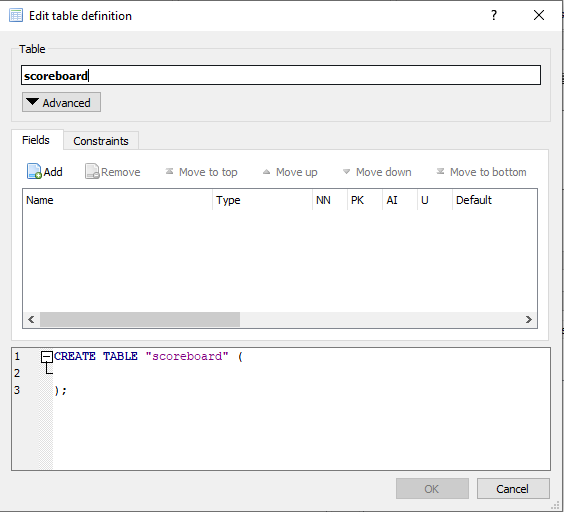
This will bring up the new database menu. From here we can go ahead and start making our first table!
Lets name it Scoreboard.
Lets start by making our id column. Click add. We are going to make it an int and lets check auto increment and non null. This will allow it to become a reference for us to pull and join data on.
Next lets create a string field and call it name. This will allow us to store the usernames for there score. Lets make the type text (used to store text up to 1tb of text data so be careful)
Finally lets go ahead and create our score. Lets add a new column and lets make the type int and name it score.
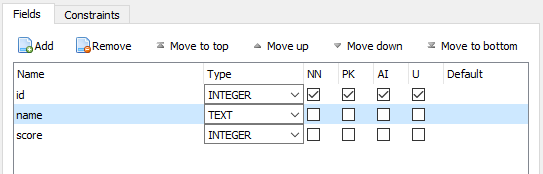

Now click ok and lets go ahead and look at our database. Go to browse data and you will see your table listed there. From here we can manually entre data if we want by click on the plus row icon and manually entering data.
If we move over to execute SQL you can see this is where we can go ahead and execute queries against our database. Lets insert some data into our table so writing in the query window lets write
insert into scoreboard (name,score) values ('nori',50),('finepointcgi',20)
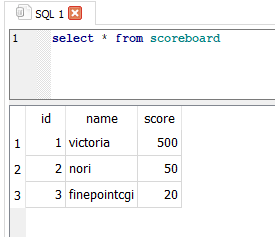

Now if we run a select statement we should see all of our data in our database.
Our database is now setup so lets move on to Godot.
Downloading the Plugin
Lets go out to the Godot and open up our asset lib and type in sqlite. We will be downloading the 2shady4u version of the SQLite plugin. So far in my testing its the best version of the Sqlite plugins I have found.
Once we have it downloaded go ahead and install it.
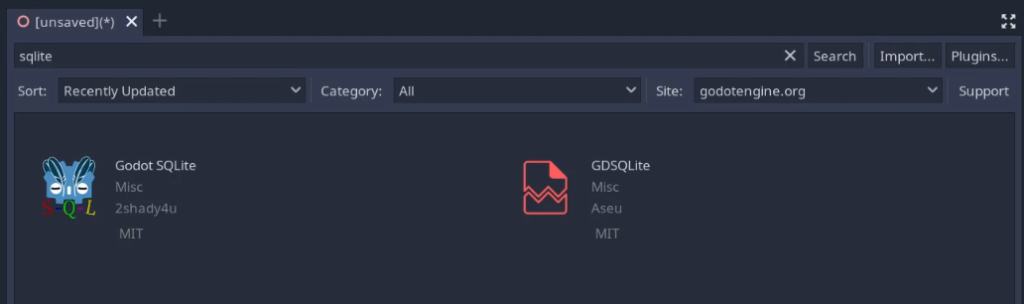
Next we will add in a Node. Then lets go ahead and create a script, lets name it MainSystem.gd. First to interface with our Godot plugin we need to load it, so at the top we will preload our data going to res://addons/godot-sqlite/bin/gdsqlite.gdns.
Inserting Data Into Our Database
const SQLite = preload("res://addons/godot-sqlite/bin/gdsqlite.gdns")
var db
var db_name = "res://DataStore/database"
func _ready():
db = SQLite.new()
db.path = db_name
func commitDataToDB():
db.open_db()
var tableName = "PlayerInfo"
var dict : Dictionary = Dictionary()
dict["Name"] = "this is a test user"
dict["Score"] = 5000
db.insert_row(tableName,dict)
We will then create our db var and our db_name var we will make it equal “res://DataStore/database”.
First we will need to initialize our database so in our ready fucntion lets call db = SQLite.new() this will set up our class to initialize our database.
After that we need to set up our database path so lets say db.path = db_name
We will also add a new function called commitDataToDB() this is where we will commit our data to our database.
First we need to create our connection so we will call db.open_db() this will open a connection for us to run querys on our databse.
We then need to pick our table name so lets make a var and call it tablename and set it to “PlayerInfo”
After this we need to create a dictionary of what information we want to insert into our database. In the dictionary the key is the column that we are inserting into the value is the value that will be inserted into our row.
Finally we will call our db.insert_row(our table name, the dictionary)
Reading Data From The Database
Like above we need to open our database and pick our table we are selecting from. We then create our query by calling db.query() and we put in our query. Once you use db.query it will fire out to query your database and return a query_result.
When then need to loop though our result. We loop though our result with our size. From here we can hook up our objects from our database. In this case we are just going to print our result.
func readFromDB():
db.open_db()
var tableName = "PlayerInfo"
db.query("select * from " + tableName + ";")
for i in range(0, db.query_result.size()):
print("Qurey results ", db.query_result[i]["Name"], db.query_result[i]["Score"])
Conclusion
So that’s pretty much it! We have added data to the database and selected it! Using databases is way easier then using files to save our data. Generally it tends to be faster and allows us to get back only the data that we need instead of reading a lot of files. Once your dataset is larger then a few files its always good to put your data into a database.