Today we’re going to be talking about sharing images and text with Android from Godot. The system works by calling the Android sub systems share system and we then pass our data to that share system and fire off our share request. The user will be prompted to share there content with an app, once they pick the app it will share it with that app eg if the app was Twitter it will tweet the content.
Getting the plugin
First we will need to download our plugin
https://github.com/Shin-NiL/Godot-Android-Share-Plugin/releases/tag/v3.0.0
Be sure to pull down the zip file. Take that zip and extract it.
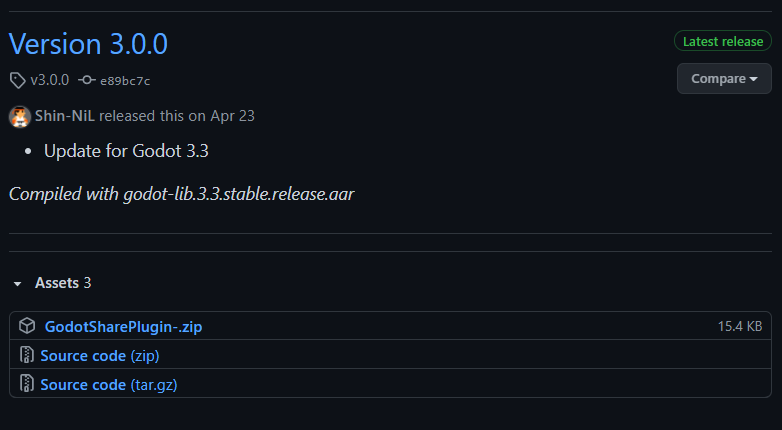
Setting up Godot
First, lets create our scene we will be doing an interface scene. From here lets create a single button. The button will be our way to share our item to the share plugin. Lets call it button and make the text “Share to Android”
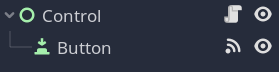
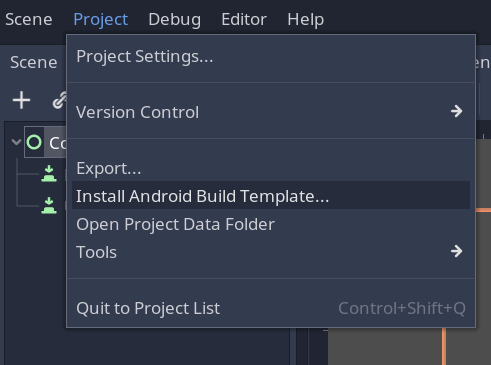
From there we need to open our Project -> Install Android Build Template. This will install the android build template. From here open up your project your file explorer and navigate over to yourproject\android\plugins and paste your extracted share library to there.
Go back into Godot and navigate to Project -> Export from there Add a preset and click Android. Click on Android and you will see on the right custom build click and enable that. Just under the custom template there is a plugins section. Enable the GodotShare plugin. From here its time to get coding!
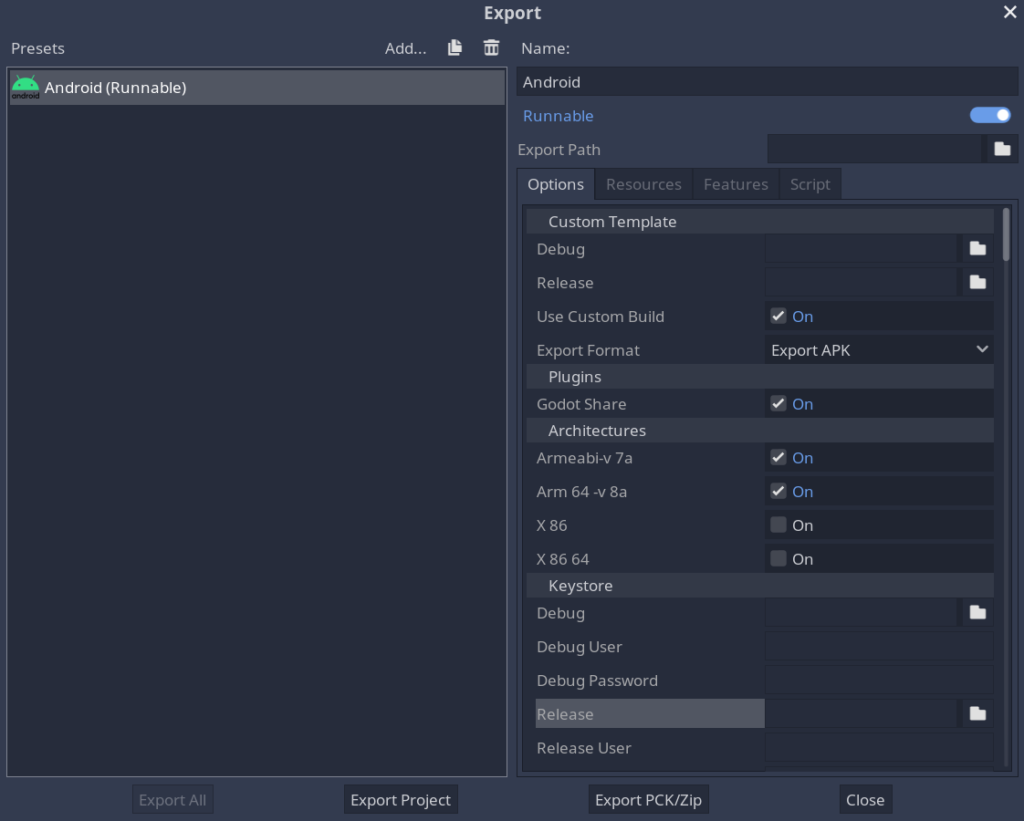
Coding The Plugin
Go to your control node and right click -> Attach Script. Lets name it Share Controller. (I do like my controllers) In our ready function we need to our Singleton its called GodotShare. You can see what it is by hovering over the plugin in the export menu. I’m going to scope my singleton outside of my ready function. This will allow me to access it everywhere.
var singleton = null
# Called when the node enters the scene tree for the first time.
func _ready():
if Engine.has_singleton("GodotShare"):
singleton = Engine.get_singleton("GodotShare")
pass
From there lets go ahead and finish setting up our button. Going out to our scene we need to connect our button signals. Click on the “button” button on the right there is a button by the inspector that says “Node” click on that, Right click button_down() -> connect, click on your control node that has your ShareController script on it and click connect. this will open up your script add the following code to your function.
func _on_ShareButton_button_down():
get_viewport().set_clear_mode(Viewport.CLEAR_MODE_ONLY_NEXT_FRAME)
yield(get_tree(), "idle_frame")
yield(get_tree(), "idle_frame")
var image = get_viewport().get_texture().get_data()
var SavedImage = OS.get_user_data_dir() + "/savedImage.png"
image.save_png(SavedImage)
singleton.sharePic(SavedImage,"shared image","Share image Godot Test", "This is my test for an upcoming #tutorial for sharing data from #Godot to #Android!")
pass # Replace with function body.
This code does a few things. First it waits until the view has cleared then it grabs a image of the viewport. It then saves an image on the user directory and fires off the sharePic call to call out to the Android Share API. The share pic function takes in a image, the title, the subject, and the body. If we want to only export text we can call:
shareText(title, subject, text)
Instead of sharePic and it will only share the text and no image.
Final Code
With this it should work! Here’s the final code!
extends Control
# Declare member variables here. Examples:
# var a = 2
# var b = "text"
var singleton = null
# Called when the node enters the scene tree for the first time.
func _ready():
if Engine.has_singleton("GodotShare"):
singleton = Engine.get_singleton("GodotShare")
pass
# Called every frame. 'delta' is the elapsed time since the previous frame.
#func _process(delta):
# pass
func _on_ShareButton_button_down():
get_viewport().set_clear_mode(Viewport.CLEAR_MODE_ONLY_NEXT_FRAME)
yield(get_tree(), "idle_frame")
yield(get_tree(), "idle_frame")
var image = get_viewport().get_texture().get_data()
var SavedImage = OS.get_user_data_dir() + "/savedImage.png"
image.save_png(SavedImage)
singleton.sharePic(SavedImage,"shared image","Share image Godot Test", "This is my test for an upcoming #tutorial for sharing data from #Godot to #Android!")
pass # Replace with function body.
Exporting The App
Next you want to export your app. If you have Godot set up its simple. (if not here’s a tutorial) First lets plugin our phone, Godot should pick up your Android phone, if it doesn’t enable developer mode on your phone, and be sure to accept the usb debugging symbols! Once Godot detects your phone you can just click the little Android guy

The app should launch. Click on the big button you made and the share drawer should open! Go ahead and click on your app. In my case im going to click on twitter.
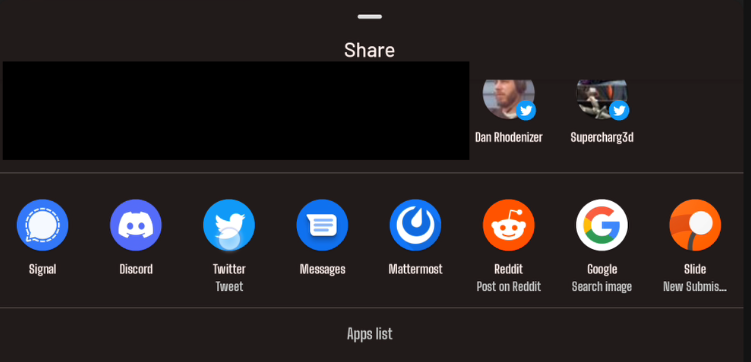
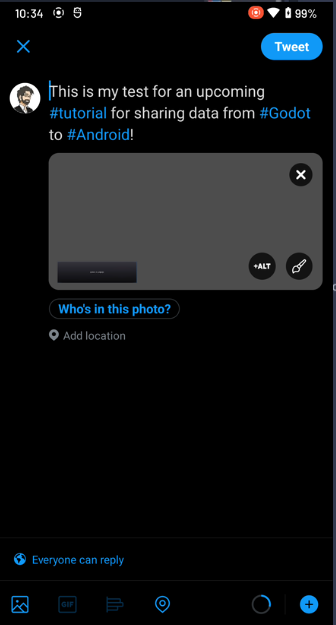
At this point it all works!
Conclusion
Well that’s all there is to it if you have any questions throw them in the comments below and ill help you. If you have any suggestions or anything that you guys want me to cover in the future also throw that in the comments below because this was a viewer suggested tutorial and I take your suggestions very seriously and ill make tutorials of things you want to see, but that’s all I have for you today, so thank you very much for reading, and ill see you next time.
Thanks