Hey Guys, lets talk about programming languages and which one you should choose
Spoiler Alert!
It doesn’t matter at all.
Mitch 2021
But we should at least talk about the advantages and disadvantages of each of the programming languages
GD Script
GD Script is a language developed by the Godot team. Its really easy to understand and easy to learn. It is very well supported by the community, most tutorials you find will be for GD script
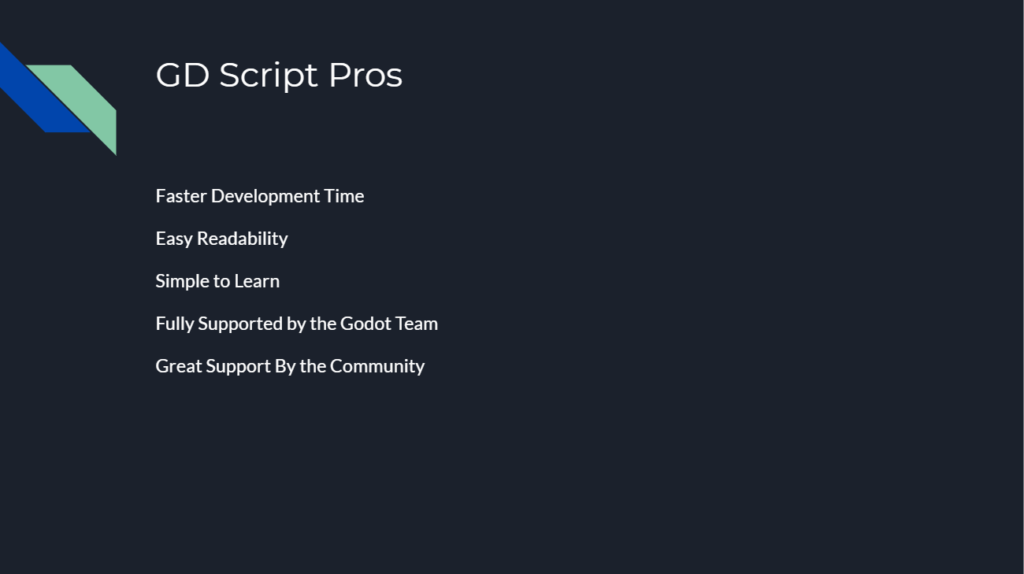
So that’s the pros what are the cons?
Its not type safe so you arnt locked down but it can be very difficult since you dont quite know exactly what your going to get back from some objects.
EG I connect to a database and get data back, what is the actual object schema no one knows with out printing or debugging it and finding out.
Its very slow I have below some benchmark results
24797 | 28001 | 26526 |
Finally it takes up more memory.
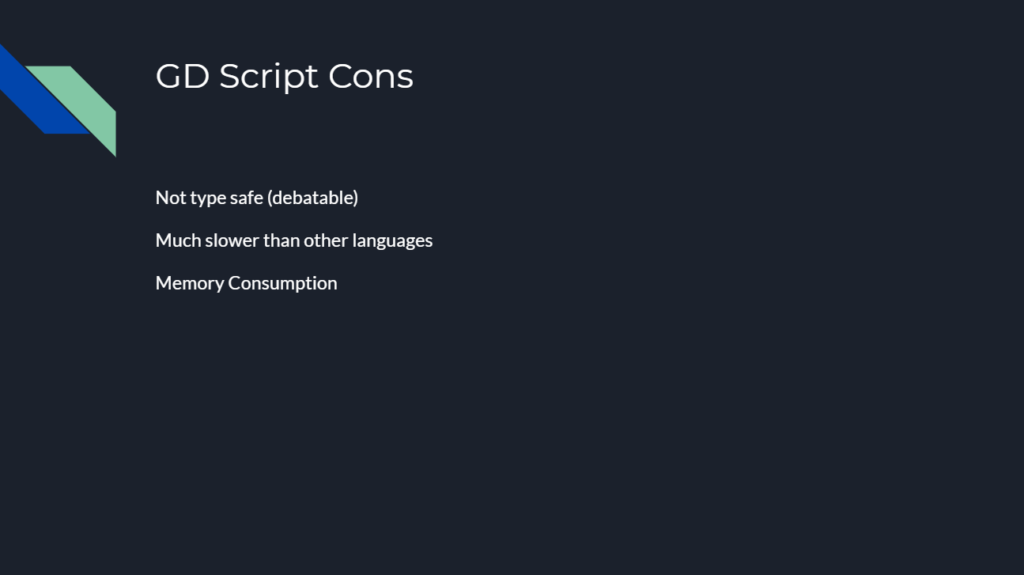
C#
Faster Performance, due to its compiled nature, the compiler makes many optimizations to your code making it much faster.
3805 | 3690 | 3621 |
In C# everything must be type safe. You know exactly what the object your getting from one function to another.
Used in other engines, C# is used in many game engines, Unity, Cryengine, Banshee, Flax. Its also used in the non-gaming industry. I use it everyday at work.
C# has thousands of libraries available to it. Unlike GD Script where you rely on people that are game developers to make an addon, you have companies and people that have been coding for years (including Microsoft) building these plugins
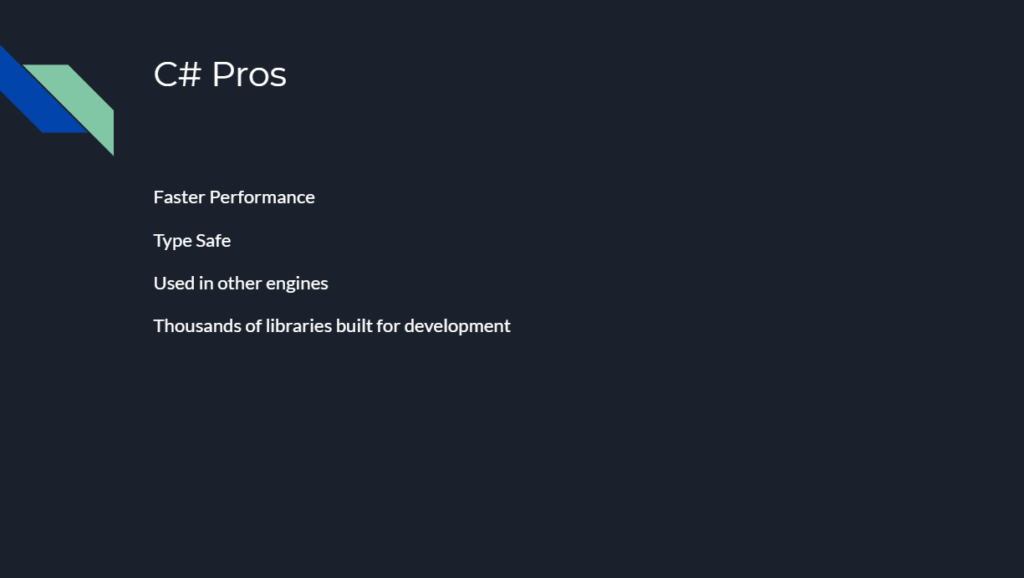
Its much more complex, being a full language there is a lot of boiler plate code that goes into each class. If you dont structure your project correctly you will have a mess on your hand.
Slow Development time – Due to the nature of C# everything has to be compiled so when you want to run your game you need to go out and compile it. This takes alot of time away from development.
Within the Godot community there is very little support and almost no tutorials/documentation so you are out in the weeds a bit. (I’m trying to make tutorials to help with this.)
Godot team does not support it very well, problems with C# is take care of slowly compared to GD script.
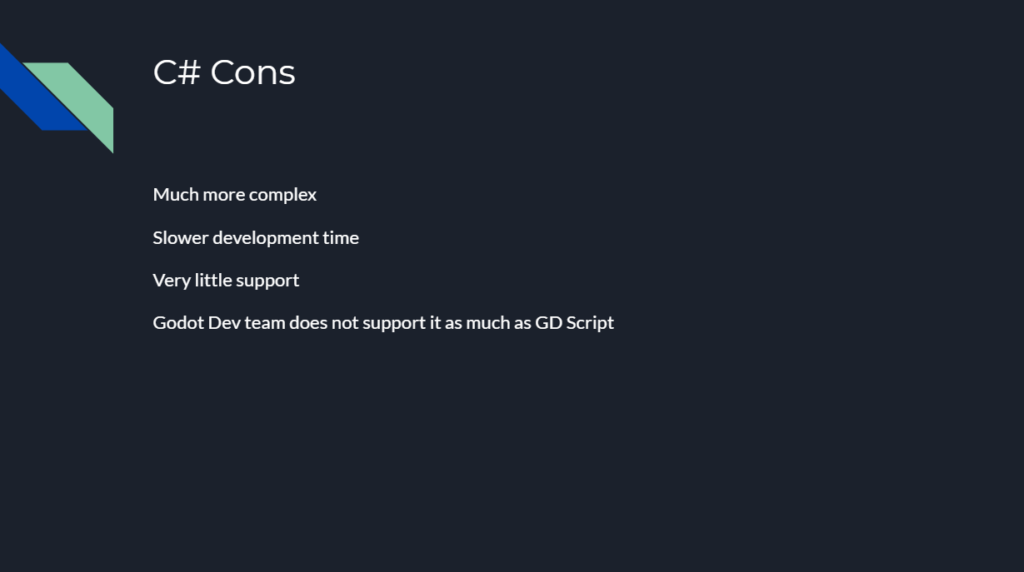
C++
Its the fastest language out there
256.11 | 293.7 | 282.74 |
Integrates directly within the Godot game engine, this allows you to directly talk to Godot’s subcomponents down to even the renderer making it faster then any other language out there.
As with this not being a custom language any C++ library with work with your C++ code, which makes it very flexible and at times quick to develop in.
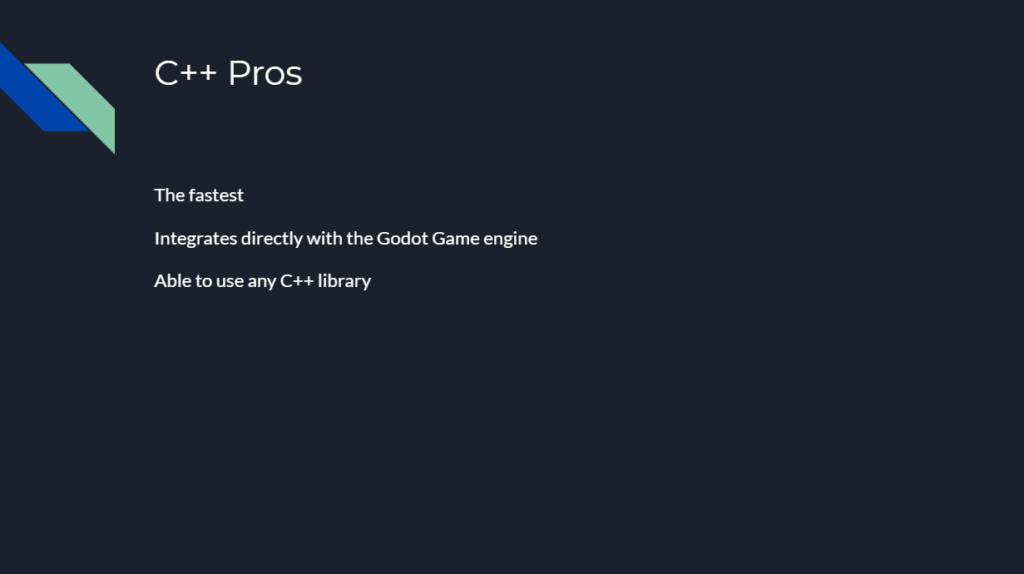
Very slow development, every time you make a change you need to compile it into a module and load it into your Godot editor for execution at runtime. This took me 2-3 hours to set up.
Really easy to be coded into a corner, with great power comes great responsibility. Using C++ you need to be careful you can easily get a memory leak or crash your computer.
Large amount of freedom, C++ allows you to touch system resources directly making you able to change even the finest detail of Godot’s game engine but sometimes walls are there to help you and prevent you from destroying your game and having unforeseen complications.
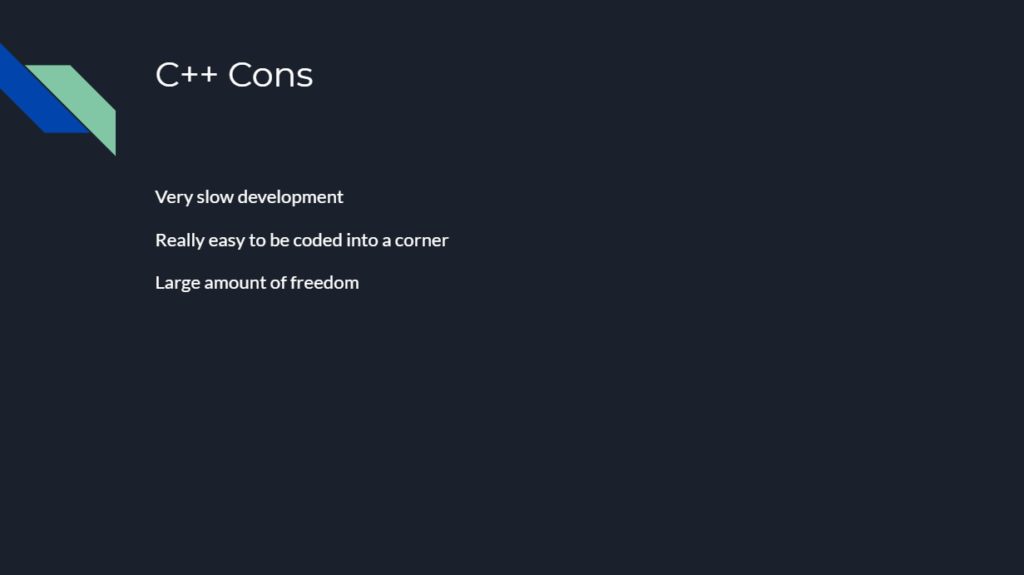
What do They Look Like?
GD Script
As you can see its very easy to read. The code is well indented and simple.
func bubbleSort(input):
var itemNeedsToMoved = true;
while (itemNeedsToMoved):
itemNeedsToMoved = false
for i in input.size() - 1:
if input[i] > input[i + 1]: var lowerValue = input[i + 1]
input[i + 1] = input[i];
input[i] = lowerValue;
itemNeedsToMoved = true;
C#
As you can see its pretty easy to read using the curly braces. The code makes good sense and its simple you just call the method and it runs though. Everything is handled by the compiler, no need to mess with a header file.
public static void BubbleSort(int[] input)
{
var itemMoved = false;
do
{
itemMoved = false;
for (int i = 0; i < input.Count() - 1; i++)
{
if (input[i] > input[i + 1])
{
var lowerValue = input[i + 1];
input[i + 1] = input[i];
input[i] = lowerValue;
itemMoved = true;
}
}
} while (itemMoved);
}
C++
This is a bit more complicated you can see GDExample is the class and int* points to the memory address of that int list. When coding you keep track of where things are in memory instead of letting the runtime handle it.
void GDExample::bubbleSort(int size, int* a)
{
for (i = 0; i < size; i++)
{
for (j = i + 1; j < size; j++)
{
if (a[j] < a[i])
{
temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
pass++;
}
}
Benchmark
As you can see GD script is 10x slower then C# and C++ is 10x faster then C#. This makes GD Script 100X slower then C++. THATS HUGE!
Run1 | Run2 | Run 3 | |
GD Script | 24797 | 28001 | 26526 |
C# | 3805 | 3690 | 3621 |
C++ | 256.11 | 293.7 | 282.74 |
Conclusion
In conclusion all languages are good, as you can see watch have a use case and you can use them in way’s that you want.
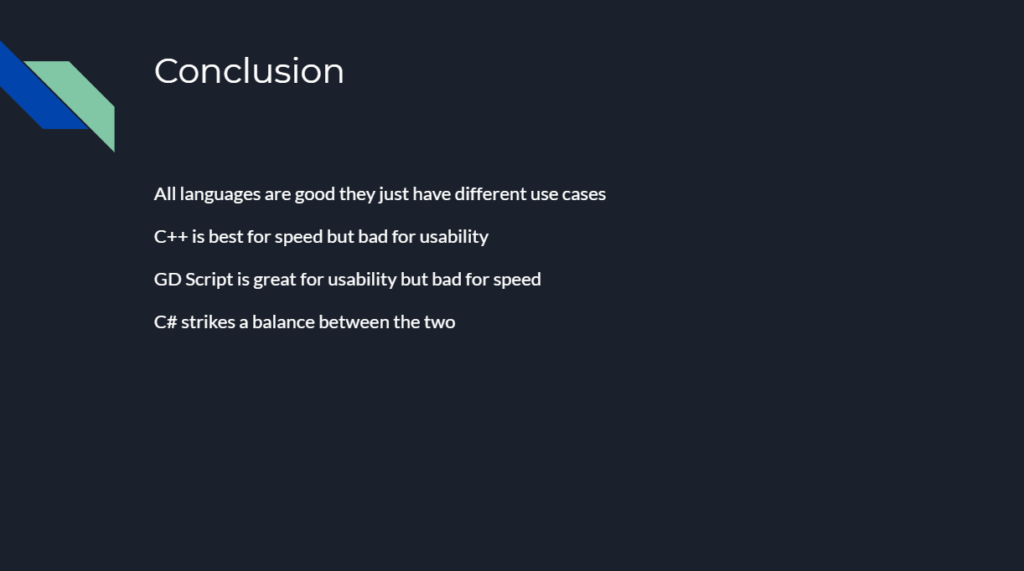
Anyway that’s all I have for you guys today! Thank you so much again for reading and ill see you all next time.
Thanks,